Learn Some Crucial Difference Between C++ and Java
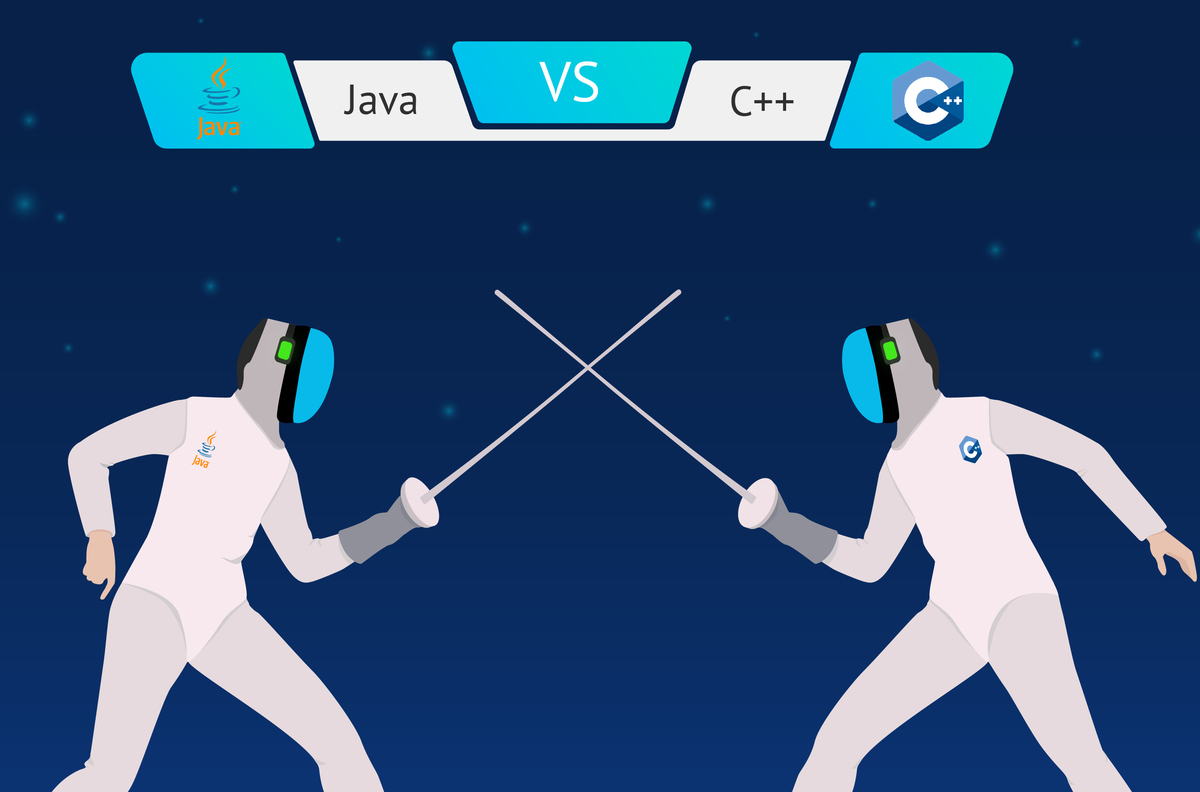
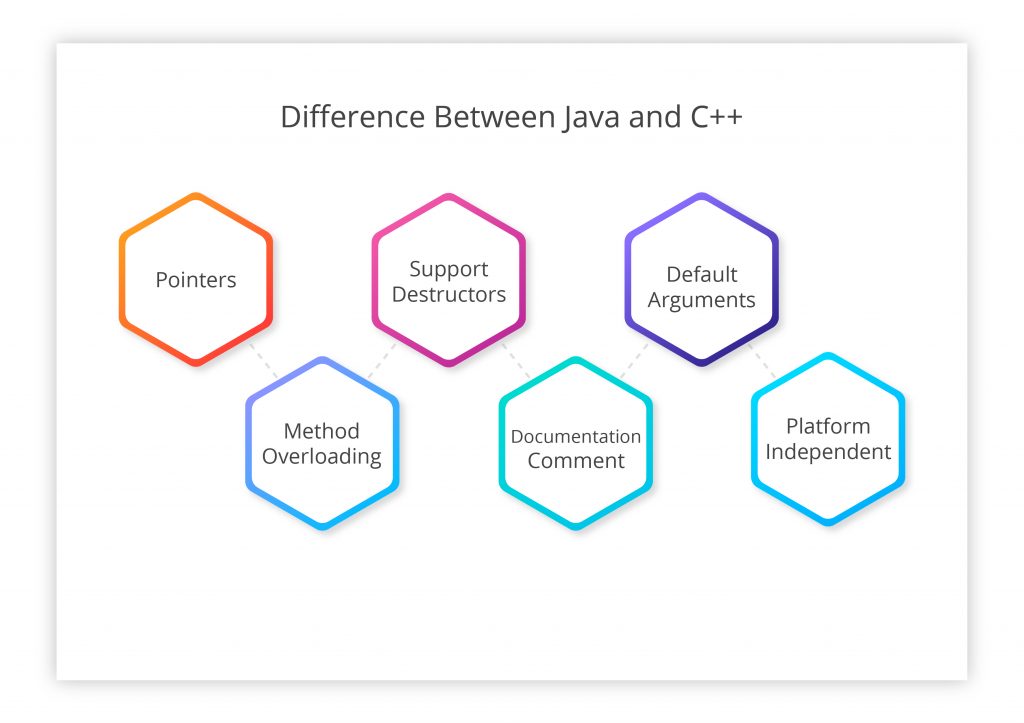
C++ programmers already have a grasp on object-oriented programming, and the syntax of Java can look familiar to them. It is understandable since Java was born out of C++. Nevertheless, there are a host of differences between C++ and Java. These differences are meant to be a C++ enhancement.
This article will attempt to contrast the performance and syntax of Java vs. C++. The awesome news is that there are many similarities between the two. This allows programmers to switch between two programming languages without much hassle.
Different design goals
The differences between Java and C++ can be traced to their virtual heritage as they have different design purposes. C++ was created as an extension of the C language. C is a procedural language. C++ is 100% object-oriented language. For instance, it has exception handling, generic programming, containers, and algorithms, etc.
Java is typed just like C++ using an object-oriented programming language (OOP). The primary motivation behind Java’s creation was to develop an easy programming language available for many platforms. The principle here was “write once, run anywhere” (WORA). It refers to the program’s ability to run on all common OSs (operating systems). Sometimes this phrase is expressed as: “write once, run everywhere” (WORE). That underpins the philosophy behind this language.
In terms of templates, C++ uses the type parameter of a class template for static methods and variables. Due to the fact that Java language uses generics, it cannot be used for static methods and variables.
Basic differences between C++ and Java
Both Java and C++ were created with object-oriented programming in mind. Yet they are different. First of all, Java is a 100% object-oriented programming language. Thus, the world of Java is a world of objects. The origin of everything is Java’s single root hierarchy. It is java.lang.Object. Conversely, C++ doesn’t have a root hierarchy, so it embraces both object-oriented and procedural programming. Thus, a fair name for it would be a “hybrid language.”
In contrast, Java is a platform-independent language. It turns the source code first into bytecode when the compilation is run. Only then this bytecode is interpreted by the interpreter and runtime is calculated to produce the output. Developers need more than one platform to develop software or run it. The most common cross-platform development language is Java. There are two ways to develop programs:
1. putting together an executable program into the operating environment (machine language and OS) of each target computer
2. using an intermediate language and compiling only once
For example, C++ applications are compiled straight to the machine language of the target computer. There’s a need to use two separate sets of source code in C++; one for Windows and the other for Macintosh.
The second method is using Java Virtual Machine as an interpreter. Java is a cross-platform language as its source code is compiled into what’s known as an intermediate “bytecode” language. Then Java Virtual Machine (JVM aka Java interpreter) executes the written bytecode for that particular hardware platform.
The Just-In-Time (JIT) compiler is part and parcel of Java Runtime Environment that enhances the performance of Java applications at run time. Java programs are comprised of classes, which include platform neutral bytecode. In its turn, JVM interprets this code on the architectures of many different computers.
The JVM loads the class files at run time, figures out the semantics of each bytecode and does the necessary computing. The JIT compiler optimizes the performance of Java programs by compiling bytecode into native machine code at run time.
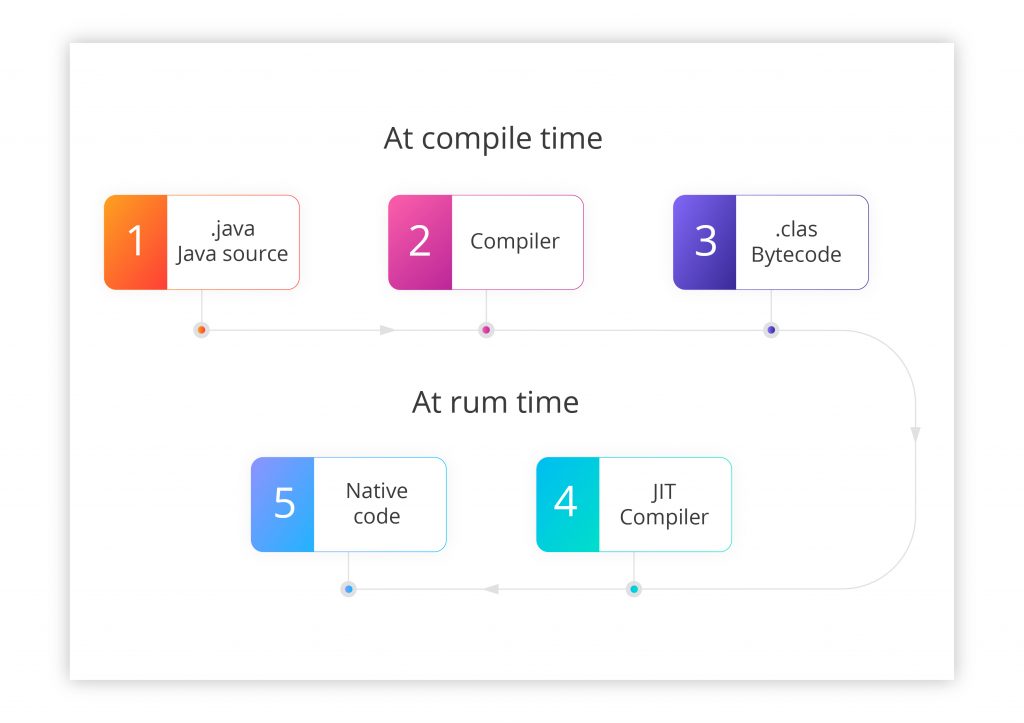
Another key difference between C++ and Java is that the latter doesn’t have destructors. In C++ every object is destroyed when it goes out of scope. For instance, a developer can declare a local object inside a function. When that function returns, the destruction of the local object happens automatically. The same happens with function parameters and also for objects returned by functions.
The object’s destructor is called right before destruction. This happens immediately. Only after that, any other program statements can be executed. Thus, there’s a certain determinism here: a C++ destructor will always execute its function. Developers can always know when and where a destructor will be executed.
Conversely, there is no such thing as a tight linkage of object destruction. There’s no calling of its finalize() method. This means objects are not explicitly destroyed in Java when going out of scope. Instead, an object is marked as unused when there aren’t any references pointing back to it. The finalize() method won’t be called until the garbage collector is on. Thus, you can never be certain as to when or where a call to finalize( ) will take place. Even if a developer executes a call to gc( ) (the garbage collector), there is no 100% guarantee that finalize( ) will automatically be executed.
In short, Java is built so that developers can write with it once and run it anywhere. And of course, it is more developer-friendly. Let’s have a look at their key differences.
Other key differences between C++ and Java
- The main difference is the Garbage Collection. Java runs all the memory by the Garbage Collector. C++ developers should keep watch of their memory management. A complex C++ application can cause computer memory shortage. In a word, it’s not easy to implement memory management in C++, as a result, there’re constant issues with memory leaks there. Garbage collection isn’t part of C++ language specification. This was done to avoid memory overhead.
- C++ uses optionally automated bounds checking (e.g., the at() method in vector and string containers). On the contrary, all operations need to be bound-checked by all compliant distributions of Java. HotSpot is used here to remove bounds checking.
- C++ is more hardware-related as opposed to Java.
- C++ has a built-in header file to incorporate diverse libraries. Import functionality is included in Java language. That encapsulates various classes and methods of this program.
- C++ uses default arguments optionally contrary to Java.
- C++ uses operator overloading.
- Java uses “pass-by-value.”
- Java doesn’t utilize “unsigned integers,” while C++ has them.
- C++ supports pointers and allows manipulating memory addresses.
- Java doesn’t support pointers making it a type-safe programming language.
- Java has Generics while C++ has templates.
- The Java Virtual Machine (JVM) helps optimize code efficiently, which contributes to a better performance of the program than in C++.
Differences in syntax
- Java syntax is created with context-free grammar. LALR parser is used to parse it. On the contrary, parsing C++ isn’t that easy. For instance, C++ uses Foo<1>(3) as a sequence to make comparisons, if there’s a “Foo variable.” Then an object is created if Foo is the name of a class template.
- In C++ objects are represented as values, while in Java language they are not. C++ uses value semantics by default, whereas, Java makes use of reference semantics. C++ can activate reference semantics either through a pointer or a reference. Java has some primitives such as int, char, long, etc.
- In C++ there’s a possibility for declaring a pointer or reference with access to a “const” object to protect client code from any modifications. Methods and functions can also guarantee they won’t alter the pointed object by a pointer via “const” keyword. This helps to keep up const-correctness.
Wrapping up
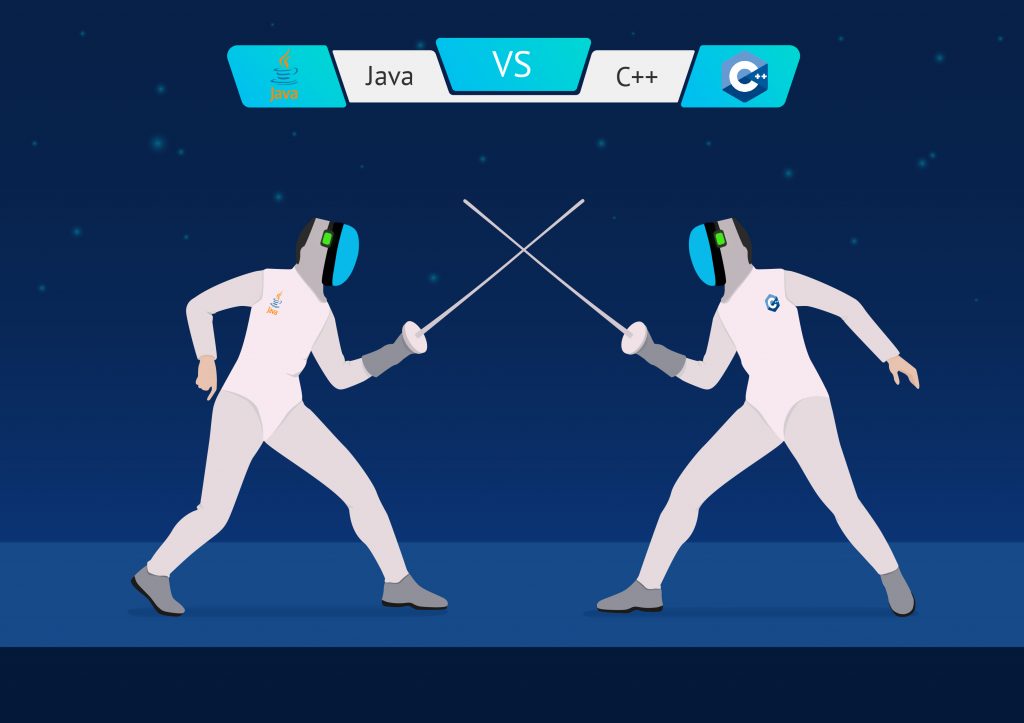
Both C++ and Java are programming languages which embrace object-oriented programming concepts. C++ gives flexibility at runtime and allows broad type hierarchies. C++ is built on the basis of C, and the former has backward compatibility with its features. It can be called a low-level language, which has some high-level features.
In C++ developers manage memory where there’s the risk of memory leakage. The developers here should have a grasp on segmentation faults. Java has an internal garbage collection system that keeps a record of allocated memory that finds paths to the objects. When the objects are not in use, then they are automatically free. Java gives access to various primitives and object types. For instance, it allows changing primitives correspondingly to their object types. They are changed into an integer object by utilizing the Integer Class Object.
Java gives room to automatic polymorphism and can limit it by banning explicit method overriding. Both C++ and Java have in-built specifiers that limit the scope of attributes and methods within one class.
Programming languages C++ and Java differ in terms of their syntax, semantics, and other areas. We encourage you to drop us a line if you would like to discuss their differences and similarities in greater detail.
Content created by our partner, Onix-systems.